Buttons
Use buttons to help the user carry out an important action such as starting a transaction or agreeing to a purchase.
-
Content:
@use 'sass:math'; @use '@ongov/ontario-design-system-global-styles/dist/styles/scss/1-variables/global.variables' as globalVariables; @use '@ongov/ontario-design-system-global-styles/dist/styles/scss/1-variables/spacing.variables' as spacing; @use '@ongov/ontario-design-system-global-styles/dist/styles/scss/1-variables/colours.variables' as colours; @use '@ongov/ontario-design-system-global-styles/dist/styles/scss/1-variables/breakpoints.variables' as breakpoints; @use '@ongov/ontario-design-system-global-styles/dist/styles/scss/1-variables/typography.variables' as typography; @use '@ongov/ontario-design-system-global-styles/dist/styles/scss/2-tools/placeholder/focus.placeholders' as placeholders; @use '@ongov/ontario-design-system-global-styles/dist/styles/scss/1-variables/font-weights.variables' as fontWeights; $ontario-button-bg-primary: colours.$ontario-colour-link; $ontario-button-bg-primary--hover: colours.$ontario-colour-link--hover; $ontario-button-bg-primary--active: colours.$ontario-colour-link--active; $ontario-button-bg-secondary: colours.$ontario-colour-white; $ontario-focus-transition: box-shadow 0.1s ease-in-out; .ontario-button { border: none; border-radius: globalVariables.$global-radius; box-sizing: border-box; box-shadow: none; display: inline-block; font-size: 1.125rem; font-family: typography.$ontario-font-open-sans; font-weight: fontWeights.$ontario-font-weights-semi-bold; line-height: calc(14 / 9); margin: spacing.$spacing-0 calc(#{spacing.$spacing-4} + #{spacing.$spacing-3}) spacing.$spacing-5 spacing.$spacing-0; min-width: 10rem; padding: math.div(spacing.$spacing-4 + spacing.$spacing-1, 2) spacing.$spacing-5; text-align: center; text-decoration: none; cursor: pointer; &:focus, &:active { @extend %ontario-focus; } @media screen and (max-width: breakpoints.$small-breakpoint) { margin-right: spacing.$spacing-0; display: block; width: globalVariables.$full-width; } .ontario-icon { margin-right: 4px; } } .ontario-button--primary { background-color: $ontario-button-bg-primary; color: colours.$ontario-colour-white; &:hover { background-color: $ontario-button-bg-primary--hover; color: colours.$ontario-colour-white; } &:focus { background-color: $ontario-button-bg-primary--hover; color: colours.$ontario-colour-white; transition: background-color 0.2s ease-out, $ontario-focus-transition; } &:active { background-color: $ontario-button-bg-primary--active; color: colours.$ontario-colour-white; transition: background-color 0s, $ontario-focus-transition; } &:visited { color: colours.$ontario-colour-white; } } .ontario-button--secondary { background-color: $ontario-button-bg-secondary; border: 2px solid colours.$ontario-colour-link; color: colours.$ontario-colour-link; padding-top: spacing.$spacing-2; padding-bottom: spacing.$spacing-2; &:hover { background-color: colours.$ontario-colour-button-secondary--hover; border-color: colours.$ontario-colour-link--hover; color: colours.$ontario-colour-link--hover; } &:focus { background-color: colours.$ontario-colour-button-secondary--hover; border-color: colours.$ontario-colour-link--hover; color: colours.$ontario-colour-link--hover; transition: background-color 0.2s ease-out, $ontario-focus-transition; } &:active { background-color: colours.$ontario-colour-button-secondary--active; border-color: colours.$ontario-colour-link--active; color: colours.$ontario-colour-link--active; transition: background-color 0s, $ontario-focus-transition; } &:visited { color: colours.$ontario-colour-link; } } .ontario-button--tertiary { background-color: transparent; color: colours.$ontario-colour-link; text-decoration: underline; &:hover { background-color: colours.$ontario-colour-button-tertiary--hover; color: $ontario-button-bg-primary--hover; text-decoration: underline; } &:focus { background-color: colours.$ontario-colour-button-tertiary--hover; color: colours.$ontario-colour-link--hover; text-decoration: underline; transition: background-color 0.2s ease-out, $ontario-focus-transition; } &:active { background-color: colours.$ontario-colour-button-tertiary--active; color: colours.$ontario-colour-link--active; text-decoration: underline; transition: background-color 0s, $ontario-focus-transition; } &:visited { color: colours.$ontario-colour-link; } }
- URL: /components/raw/buttons/buttons.scss
- Filesystem Path: fractal/components/components/buttons/buttons.scss
- Size: 4.4 KB
-
Content:
.ontario-button:focus,.ontario-button:active{box-shadow:0 0 0 4px #009adb;outline:4px solid transparent;transition:box-shadow .1s ease-in-out}.ontario-button{border:none;border-radius:4px;box-sizing:border-box;box-shadow:none;display:inline-block;font-size:1.125rem;font-family:"Open Sans","Helvetica Neue",Helvetica,Arial,sans-serif;font-weight:600;line-height:1.5555555556;margin:0 calc(1rem + 0.75rem) 1.5rem 0;min-width:10rem;padding:.625rem 1.5rem;text-align:center;text-decoration:none;cursor:pointer}@media screen and (max-width: 40em){.ontario-button{margin-right:0;display:block;width:100%}}.ontario-button .ontario-icon{margin-right:4px}.ontario-button--primary{background-color:#06c;color:#fff}.ontario-button--primary:hover{background-color:#00478f;color:#fff}.ontario-button--primary:focus{background-color:#00478f;color:#fff;transition:background-color .2s ease-out,box-shadow .1s ease-in-out}.ontario-button--primary:active{background-color:#002142;color:#fff;transition:background-color 0s,box-shadow .1s ease-in-out}.ontario-button--primary:visited{color:#fff}.ontario-button--secondary{background-color:#fff;border:2px solid #06c;color:#06c;padding-top:.5rem;padding-bottom:.5rem}.ontario-button--secondary:hover{background-color:#e0f0ff;border-color:#00478f;color:#00478f}.ontario-button--secondary:focus{background-color:#e0f0ff;border-color:#00478f;color:#00478f;transition:background-color .2s ease-out,box-shadow .1s ease-in-out}.ontario-button--secondary:active{background-color:#c2e0ff;border-color:#002142;color:#002142;transition:background-color 0s,box-shadow .1s ease-in-out}.ontario-button--secondary:visited{color:#06c}.ontario-button--tertiary{background-color:transparent;color:#06c;text-decoration:underline}.ontario-button--tertiary:hover{background-color:#e8e8e8;color:#00478f;text-decoration:underline}.ontario-button--tertiary:focus{background-color:#e8e8e8;color:#00478f;text-decoration:underline;transition:background-color .2s ease-out,box-shadow .1s ease-in-out}.ontario-button--tertiary:active{background-color:#d1d1d1;color:#002142;text-decoration:underline;transition:background-color 0s,box-shadow .1s ease-in-out}.ontario-button--tertiary:visited{color:#06c}
- URL: /components/raw/buttons/buttons.css
- Filesystem Path: fractal/components/components/buttons/buttons.css
- Size: 2.2 KB
Guiding principle: Be consistent.
When to use this component
Use buttons when you want the user to do something (often called a “call to action”), for example:
- start an application process or a transaction
- agree to make a payment
- download a PDF
Don’t use a button when you’re not encouraging an action. Buttons should not be used the same way as links, which usually send the user to another page or to a different part of the same page.
Types of buttons
Use a primary button to draw attention to the main action you want to help the user take. Avoid using multiple primary buttons on one page unless the page has equally important calls to action.
Use a secondary button for a less important call to action on a page. Avoid using multiple secondary buttons so the user is not confused about what they should do next. Instead, ask the writer to simplify or break up the content so that it doesn’t need multiple secondary buttons.
Use tertiary buttons for links that should function like a button, such as “edit” or “cancel” in applications. It’s okay to use more than one tertiary button on a page.
Disabled buttons
Disabling buttons until users have correctly completed a form can cause usability and accessibility problems. Disabled buttons can create barriers for assistive technology users and users with physical or cognitive impairments, and they can be confusing and frustrating for all users because they do not help the user understand what they need to do to proceed.
A better approach is to keep buttons enabled and instead develop error handling to provide clear feedback about any missed fields or input errors when the user tries to submit the form. For guidance on error messaging, see Page alerts.
Technical specifications
Button type | Colour | Minimum width |
---|---|---|
Primary button | Default background: #0066CC Font: #FFFFFF Hover background: #00478F Active background: #002142 |
160px |
Secondary button | Default background: #FFFFFF Default border and font: #0066CC Hover background: #E0F0FF Hover border and font: #00478F Active background: #C2E0FF Active border and font: #002142 |
160px |
Tertiary button | Default background: none Default border: none Default font: #0066CC Hover background: #E8E8E8 Hover font: #00478F Active background: #D1D1D1 Active font: #002142 |
160px |
The width of primary, secondary and tertiary buttons (minimum 160px) should be the size of the text label with additional padding:
- 24px internal padding on the right and left sides of the button text
- 16px internal padding on the top and bottom of the button text
There should be 32px spacing between buttons on desktop and 24px spacing between buttons on mobile.
Desktop
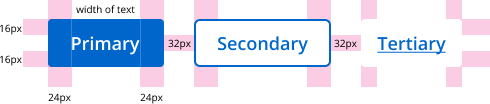
Mobile
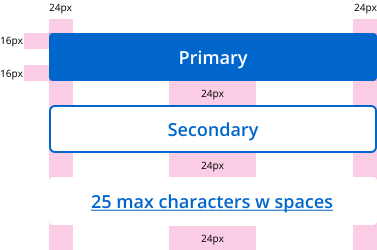
Do:
- ensure button text is clear, descriptive and action-oriented (for example, “Save your profile” or “Download your receipt”)
- use sentence case (capitalize only the first letter of the first word)
- use a maximum of 25 characters including spaces
- place buttons below form elements
- when reading online, people’s eyes typically follow an “F” pattern, so you’re making it easier for the user by putting the call-to-action in a button where their eyes are naturally drawn anyway
The “F” pattern
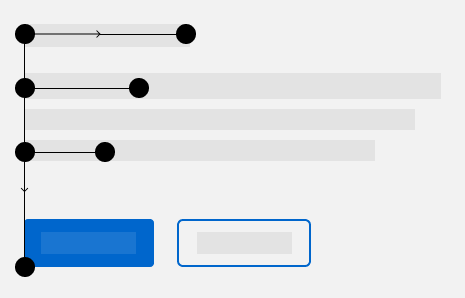
Don’t:
- change button colours
- underline text for primary or secondary buttons
- use multiple primary and secondary buttons unless absolutely necessary
- tertiary buttons aren’t as prominent as primary or secondary buttons so you can use a few if needed
If you have any questions or feedback, please get in touch.