Radio buttons
Use radio buttons when you want the user to select only one option from a list.
-
Content:
@use 'sass:math'; @use '@ontario-digital-service/ontario-design-system-global-styles/dist/styles/scss/1-variables/global.variables' as globalVariables; @use '@ontario-digital-service/ontario-design-system-global-styles/dist/styles/scss/1-variables/spacing.variables' as spacing; @use '@ontario-digital-service/ontario-design-system-global-styles/dist/styles/scss/1-variables/colours.variables' as colours; @use '@ontario-digital-service/ontario-design-system-global-styles/dist/styles/scss/1-variables/breakpoints.variables' as breakpoints; @use '@ontario-digital-service/ontario-design-system-global-styles/dist/styles/scss/1-variables/typography.variables' as typography; @use '@ontario-digital-service/ontario-design-system-global-styles/dist/styles/scss/1-variables/z-index.variables' as zIndex; @use '@ontario-digital-service/ontario-design-system-global-styles/dist/styles/scss/1-variables/font-sizes.variables' as fontSizes; $ontario-touch-target-size: 36px; $ontario-touch-target-size-mobile: 40px; $ontario-radios-size: 32px; $ontario-radios-size-mobile: 36px; $ontario-input-offset: math.div($ontario-touch-target-size - $ontario-radios-size, 2); * .ontario-radios { -webkit-tap-highlight-color: rgba(0, 0, 0, 0); -moz-tap-highlight-color: rgba(0, 0, 0, 0); } .ontario-radios { padding: 0 0 0 math.div(spacing.$spacing-1, 2); margin-bottom: spacing.$spacing-7; max-width: globalVariables.$standard-width; } .ontario-radios__item { position: relative; display: block; margin: spacing.$spacing-0 spacing.$spacing-0 spacing.$spacing-4; padding: spacing.$spacing-0 spacing.$spacing-6 spacing.$spacing-0; &:last-of-type { margin-bottom: spacing.$spacing-0; } } .ontario-radios__input { cursor: pointer; margin: spacing.$spacing-0; position: absolute; opacity: 0; top: $ontario-input-offset * -1; left: $ontario-input-offset * -1; width: $ontario-touch-target-size; height: $ontario-touch-target-size; z-index: zIndex.$ontario-z-index-above-low; @media screen and (max-width: breakpoints.$small-breakpoint) { width: $ontario-touch-target-size-mobile; height: $ontario-touch-target-size-mobile; top: $ontario-input-offset * -0.75; left: $ontario-input-offset * -0.75; } } .ontario-radios__label { display: inline-block; font-size: fontSizes.$ontario-font-size-standard-body-text; margin: 0.2rem spacing.$spacing-0; padding: spacing.$spacing-0 spacing.$spacing-3; // remove 300ms pause on mobile touch-action: manipulation; white-space: normal; @media screen and (max-width: breakpoints.$small-breakpoint) { margin: 0.3rem spacing.$spacing-0; padding: 0 1rem; } &:before { content: ''; border: 2px solid colours.$ontario-colour-black; border-radius: 50%; box-sizing: border-box; position: absolute; top: 0; left: 0; width: $ontario-radios-size; height: $ontario-radios-size; transition: border 0.1s ease-in-out; @media screen and (max-width: breakpoints.$small-breakpoint) { width: $ontario-radios-size-mobile; height: $ontario-radios-size-mobile; } } &:after { content: ''; border-radius: 50%; border: 10px solid colours.$ontario-colour-black; position: absolute; top: 0; left: 0; transform: translate($ontario-input-offset * 3, $ontario-input-offset * 3); opacity: 0; @media screen and (max-width: breakpoints.$small-breakpoint) { border: 12px solid colours.$ontario-colour-black; } } } .ontario-radios__input:focus + .ontario-radios__label:before { -moz-box-shadow: 0 0 0 4px colours.$ontario-colour-focus; -webkit-box-shadow: colours.$ontario-colour-focus; box-shadow: 0 0 0 4px colours.$ontario-colour-focus; outline: 4px solid transparent; transition: box-shadow 0.1s ease-in-out; } .ontario-radios__input:checked + .ontario-radios__label:after { opacity: 1; }
- URL: /components/raw/radio-buttons/radio-buttons.scss
- Filesystem Path: fractal/components/components/form/radio-buttons/radio-buttons.scss
- Size: 3.8 KB
-
Content:
* .ontario-radios{-webkit-tap-highlight-color:rgba(0,0,0,0);-moz-tap-highlight-color:rgba(0,0,0,0)}.ontario-radios{padding:0 0 0 .125rem;margin-bottom:2.5rem;max-width:48rem}.ontario-radios__item{position:relative;display:block;margin:0 0 1rem;padding:0 2rem 0}.ontario-radios__item:last-of-type{margin-bottom:0}.ontario-radios__input{cursor:pointer;margin:0;position:absolute;opacity:0;top:-2px;left:-2px;width:36px;height:36px;z-index:1}@media screen and (max-width: 40em){.ontario-radios__input{width:40px;height:40px;top:-1.5px;left:-1.5px}}.ontario-radios__label{display:inline-block;font-size:1rem;margin:.2rem 0;padding:0 .75rem;touch-action:manipulation;white-space:normal}@media screen and (max-width: 40em){.ontario-radios__label{margin:.3rem 0;padding:0 1rem}}.ontario-radios__label:before{content:"";border:2px solid #1a1a1a;border-radius:50%;box-sizing:border-box;position:absolute;top:0;left:0;width:32px;height:32px;transition:border .1s ease-in-out}@media screen and (max-width: 40em){.ontario-radios__label:before{width:36px;height:36px}}.ontario-radios__label:after{content:"";border-radius:50%;border:10px solid #1a1a1a;position:absolute;top:0;left:0;transform:translate(6px, 6px);opacity:0}@media screen and (max-width: 40em){.ontario-radios__label:after{border:12px solid #1a1a1a}}.ontario-radios__input:focus+.ontario-radios__label:before{box-shadow:0 0 0 4px #009adb;outline:4px solid transparent;transition:box-shadow .1s ease-in-out}.ontario-radios__input:checked+.ontario-radios__label:after{opacity:1}
- URL: /components/raw/radio-buttons/radio-buttons.css
- Filesystem Path: fractal/components/components/form/radio-buttons/radio-buttons.css
- Size: 1.5 KB
Guiding principle: Give the user control.
When to use this component
Use radio buttons when you want the user to select only one option from a list.
If you want the user to select more than one option use a list with checkboxes.
Order of radio button lists
Put radio button lists in alphabetical order, with these exceptions:
- list yes before no
- if there is an option that you know about 90% or more of your users will choose, put that option first
- for example, list Ontario first if users are choosing their province from a list
- be careful not to bias the user’s choice or imply the importance of option one over another
- put any “None of the above” or “I do not know” options last
Technical specifications
Example
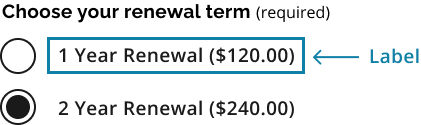
Do:
- always include an associated label with a matching ID next to each radio button
- position radio buttons to the left of their labels
- left-align radio buttons
- always make the label and the radio button selectable
- stack radio buttons vertically
- exception: yes/no can be listed horizontally
- include a “None of the above” or “I do not know” option if they are valid because users cannot go back to having no option selected once they have selected one
Don’t:
- change a radio button’s default size (32px on desktop and 36px on mobile, with a hit area of 48px for both)
- pre-select radio buttons (users might miss the question or submit the wrong answer)
If you have any questions or feedback, please get in touch.